“ 지연되는 프로젝트에 인력을 더 투입하면 오히려 더 늦어진다. ”
블로그를 시작하면서 선생님과 약속한 1일 1블로그에 위기가 찾아왔어요....
주제가 없는데 글을 올려야 하는 상황이 오고말았어요....
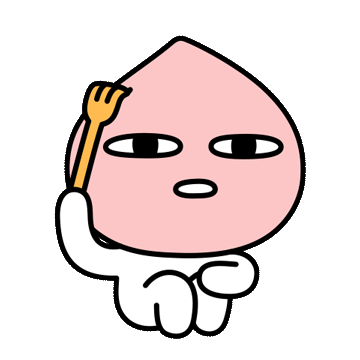
뭘 적어야 하는가 생각해봤는데
오늘은 명확한 주제를 가지고 정리하는 것 말고 오늘 수업에서 공부한 걸 정리하려고 해요...
앞으로도 여러번 쓸거같은 예감이ㅎㅎ
형변화
그저께 올린 문제중 형과 관련된 함수가 있었는데
parseInt() : 문자열을 정수로 바꾸는 함수(숫자가 아닐 경우 nan을 반환함)
isNaN() : 매개변수가 숫자인지 검사하는 함수
ispositive() : 양수인지 확인하는 함수
이렇게 세가지 였습니다.
이렇게 세가지 함수와 더불어 오늘은 형변화에 대해 수업이 먼저 이루어졌습니다.
형변화를 배우기 전에 type of 함수로 형을 판별하는 것부터 배웠습니다.
let x= 100, y = "200", z= undefined;
const func = function(){}
document.write(x,"<br>");
document.write(typeof(x),"<br>"); // 타입 판별
//*******데이터 저장하기에
document.write(y,"<br>");
document.write(typeof(y),"<br>"); // 타입 판별
document.write(z,"<br>");
document.write(typeof(z),"<br>"); // 타입 판별
document.write(func,"<br>");
document.write(typeof(func)); // 타입 판별
결과 100 number 200 string undefined undefined function(){} function |
위와 같이 각각의 값들의 데이터형들을 판별하여 출력할때 사용하는 함수 입니다.
typeof함수를 토대로 형변화를 해보면
let y = "200"
document.write(y,"<br>");
document.write(typeof(y),"<br>"); // 타입 판별
y= Number(y,"<br>"); // 타입 변경
document.write(typeof(y),"<br>"); // 타입 판별
먼저 위에서 200이 string(문자)로 출력되었지만 num()함수를 통해 y의 값을 숫자로 출력한 것을 볼 수있습니다.
이처럼 데이터형을 변형시켜 코딩에 유용하게 사용될 수 있다는 것을 배웠습니다.
데이터 불러오기
형변화가 끝난 후 예전에 배우던 데이터 불러오기를 더 보충하여 공부하였습니다.
forEach()문
주로 우리가 많이 사용하는 for문에는 단점이 하나 존재합니다.
그건 비슷한 조건문을 자주 많이 사용한다는 것입니다.
매번 조건식과 실행문을 사용하기 귀찮은데 이럴 때 이것을 보안한 것이 forEach문입니다.
배열을 for문으로 출력할때 기본적으로 가지고있는 내장함수를 사용할 수있는데 그것이 forEach메소드입니다.
심지어 화살표함수로 간략하게 사용할 수있고 괄호까지 생락해서 간략하게 사용할 수있습니다.
똣한 element(요소-배열의 값), index(인덱스-배열의 자리값), array(배열)을 한번에 출력할 수 있습니다.
let num = [100,200,300,400,500];
// forEach
num.forEach(function(el){ //element 약어
document.write(el,"");
});
document.write("");
// forEach : 화살표
num.forEach((el) => {
document.write(el,"");
});
document.write("");
// forEach : 화살표 : 괄호 생략
num.forEach(el => {
document.write(el,"");
});
document.write("");
// forEach : 화살표 : 괄호 생략
num.forEach(el => document.write(el,""));
document.write("");
// forEach(값, 인덱스, 배열)
num.forEach(function(element, index, array){
document.write(element,"");
document.write(index,"");
document.write(array,"");
});
100 ~ 500 100 ~ 500 100 ~ 500 100 ~ 500 100 0 100,200,300,400,500 ~ 500 4 100,200,300,400,500 |
for of 문
가장 우리가 많이 사용하던 반복문의 다른 모습입니다.
for of문은 배열에 가지고 있는 요소들을 모조리 다 꺼내는 문법으로 인덱스는 출력을 할 수 없습니다.
const arr = [100,200,300,400,500];
for(let i of arr){
document.write(i); // forEach보다 짧고 쉽게 출력
};
100 200 300 400 500 |
for in문
선언된 객체의 모든 키와값을 꺼내서 출력할 수 있는 문법입니다.
for of와 다른점은 객체를 위해 만들어진 문법으로 키값을 출력할수 있습니다.
하지만 배열에도 사용가능하며 이는 객체가 배열에서 파생된 것이므로 배열에도 사용이 가능하지만 주로 객체에 많이 사용하는 문법입니다.
const arr = [100,200,300,400,500];
for(let i in arr){
document.write(i); // 인덱스 값 출력
};
document.write("");
for(let i in arr){
document.write(arr[i]); // 값 출력
};
01234 //인덱스값 100200300400500 // 요소값 |
map()
map()함수는 실행결과를 가지고 새로운 배열을 만들 때 사용합니다.
오늘은 새로운 배열을 만들지는 않고 출력하는 것을 해보았습니다.
const num = [100,200,300,400,500];
num.map(function(el, i, a){
console.log(el) // 요소
console.log(i) // 인덱스
console.log(a) // 배열
});
100 // 요소(값) 0 // 인덱스 (5) [100, 200, 300, 400, 500] // 배열 200 1 (5) [100, 200, 300, 400, 500] 300 2 (5) [100, 200, 300, 400, 500] 400 3 (5) [100, 200, 300, 400, 500] 500 4 (5) [100, 200, 300, 400, 500] |
함수 시험
늘 시험을 보지만 오늘은 진짜 힘들었던거 같아요 아무리해도 함수가 머리에 남질 않아서...
일단 외워서 시험 보려고 합니다.
화이팅...
시험 내용였습니다.
//01. 선언적함수 (함수가 실행되었습니다)
{
function func(){
document.write("01. 함수가 실행되었습니다.");
}
func();
}
document.write("<br>");
document.write("<br>");
//02. 익명함수
{
const func = function(){
document.write("02. 함수가 실행되었습니다.");
}
func();
}
document.write("<br>");
document.write("<br>");
//03. for문 함수10번
{
function func(){
for(let i=1; i<=10; i++){
document.write("03. 함수가 실행되었습니다.<br>");
}
}
func();
}
document.write("<br>");
document.write("<br>");
//04. 리턴값함수
{
function func(){
const a = "04. 함수가 실행되었습니다."
return a;
}
document.write(func());
}
document.write("<br>");
document.write("<br>");
//05. 매개변수함수
{
function func(a){
document.write(a);
}
func("05. 함수가 실행되었습니다");
}
document.write("<br>");
document.write("<br>");
//06. 선언적함수 화살표
{
func = () => {
document.write("06. 함수가 실행되었습니다.");
}
func();
}
document.write("<br>");
document.write("<br>");
//07. 10칸테이블
{
function func(){
let table = "<table border='1'>"
let num = 1;
for(let i=1; i<=10; i++){
table += "<tr>";
for(let j=1; j<=10; j++){
table += "<td>"+num+"</td>";
num++
}
table += "</tr>"
}
table += "</table>";
document.write(table);
}
func();
}
이 밖에 디자인을 코딩해보는 것도 해봤는데 그건 완성되면 올리도록 하겠습니다.
그럼 오늘 이만 마무리 하겠습니다.
모두 화이팅!